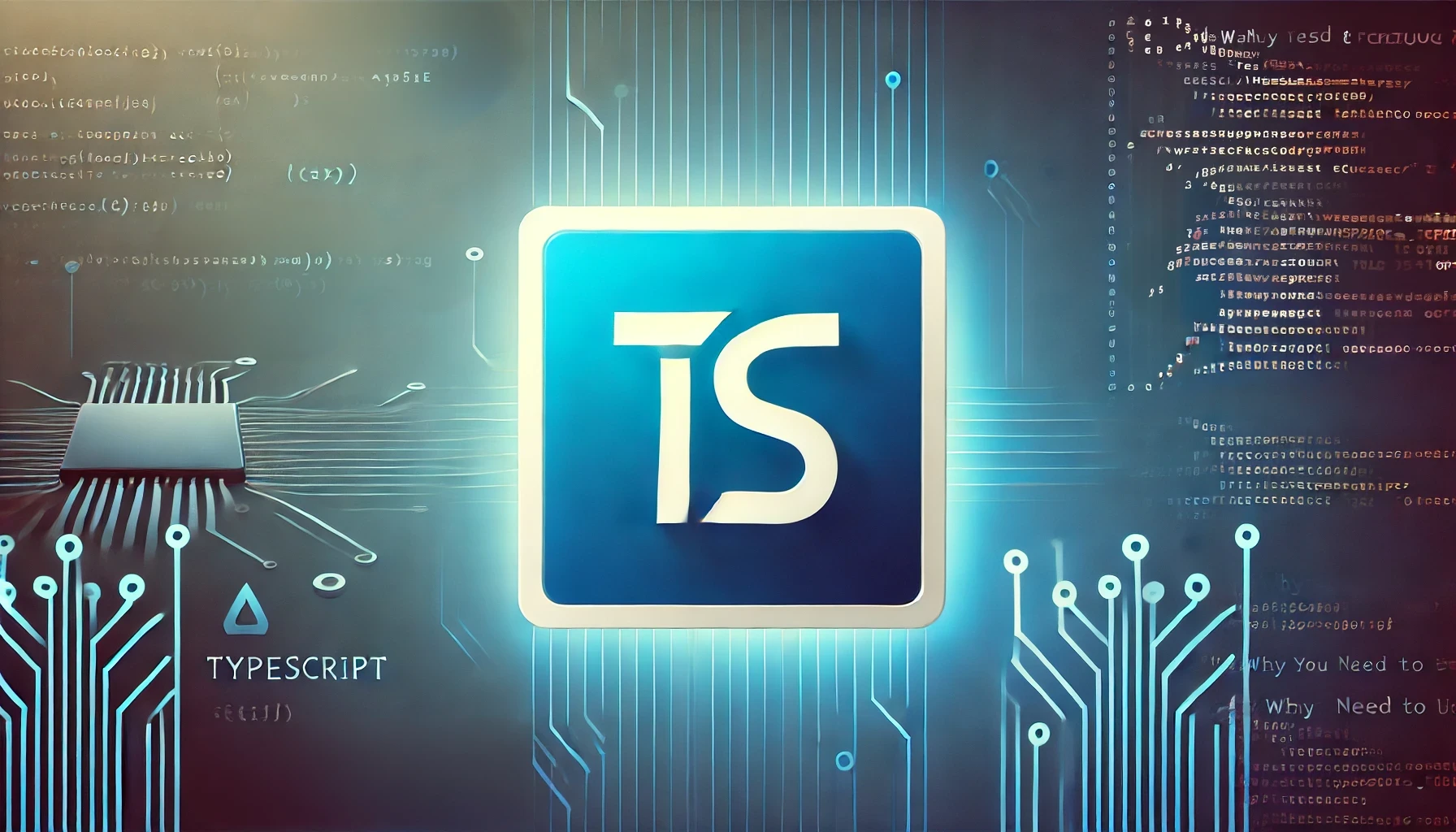
Why you need to use Typescript
What is Typescript?
Typescript is a statically typed superset of javascript that can be compiled to plain javascript.
Statically typed: All variables have a type such as string, number, boolean, etc. Once the type has been defined it cannot be changed without typescript getting mad.
Superset of javascript: The added syntax is completely optional because all javascript is typescript. You can just write plain javascript without learning any new syntax and reap the benefits of type checking due to the implicit types inferred by the compiler.
Compiles to javascript: The additional syntax in typescript is not understood by browsers or node so a compiler is used to convert the typescript specific syntax to plain javascript. You can then run the javascript anywhere you would normally run javascript like in the browser or on a server with node. (Although newer JS runtimes such as deno and bun support typescript directly without a compiler).
TLDR: Its javascript with types.
Why use Typescript?
Popularity: Just looking at the number of package downloads from npm compared to react, typescript has over double the amount of downloads according to npm trends:
According to the state of js survey less than 10% of developer are writing 100% javascript. Most are using some amount of typescript in their projects:
Code quality: Using typescript increases the quality and understandability of your code. Defining types provides documentation to future developers on the intent of a variable or function.
Reduced Bugs: Its is better to find issues at compile time rather than runtime. Typescript allows you to solve a lot of common javascript problems when compiling as oppsed to running your application.
Refactorable: Types make refactoring code easier. How many times have you had working code, changed something, and then no longer had working code? This happens all the time. Typescript can help you more easily find where your code is breaking.
How does javascript handle typing?
Javascript’s approach to typing is often refered to as “duck typing”. If it looks like a duck and quacks like a duck then it is probably a duck. In javascript if it looks like a string and is being treated like a string then javascript will assume its a string.
Whats the problem with that? Well the way javascript can handle types can be hard to predict leading to weird edge cases.
For example you may assume a + b
is the same thing as b + a
but that is not always the case in javascript. Just switching the order produces different results which can be non-intuitive:
ts
[] + {} // '[object Object]'{} + [] // 0
Lets consider another basic logic princaipal. If a == b
and b == c
then a == c
… Not in javascript:
ts
0 == [] // true0 == "0" // true"0" == [] // false
The common thing between these two examples is the use of diferent types together forcing javascript to make some assuptions about the developers intentions.
Typescript forces the developer to be explicit about their intentions and by default does not allow you to mix types in these ways preventing a lot of issues.
TLDR: Typescript makes code more intuitive and predictable than javascript.
Common isses solved by typescript
Here are some examples of issues that can be solved with type script. Look at the javascript and see if you can predict whtat the output will be. You can check yourself by looking at the output tab. Click the typescript tab to see how typescript would handle these scenarios
Example 1
ts
const rectangle = {width: 10,height: 15,};const area = rectangle.width * rectangle.heigth;console.log(`Area: ${area}`);
ts
constrectangle = {width : 10,height : 15,};constProperty 'heigth' does not exist on type '{ width: number; height: number; }'. Did you mean 'height'?2551Property 'heigth' does not exist on type '{ width: number; height: number; }'. Did you mean 'height'?area =rectangle .width *rectangle .; heigth console .log (`Area: ${area }`);
bash
$ node area.jsArea: NaN
See Explanation
Javascript will let you reference properties and methods that do not exists. This is most commonly seen with typos. Javascript will not warn you if you make a typo but typesript will.
Example 2
ts
import readline from "readline-sync";var grade = readline.question("Grade: ");if (90 < grade < 100) {console.log("A: Pass");} else if (80 < grade < 90) {console.log("B: Pass");} else if (70 < grade < 80) {console.log("C: Pass");} else if (60 < grade < 70) {console.log("D: Pass");} else {console.log("F: Fail");}
ts
importreadline from "readline-sync";vargrade =readline .question ("Grade: ");if (90 <Operator '<' cannot be applied to types 'boolean' and 'number'.2365Operator '<' cannot be applied to types 'boolean' and 'number'.grade < 100) {console .log ("A: Pass");} else if (80 <Operator '<' cannot be applied to types 'boolean' and 'number'.2365Operator '<' cannot be applied to types 'boolean' and 'number'.grade < 90) {console .log ("B: Pass");} else if (70 <Operator '<' cannot be applied to types 'boolean' and 'number'.2365Operator '<' cannot be applied to types 'boolean' and 'number'.grade < 80) {console .log ("C: Pass");} else if (60 <Operator '<' cannot be applied to types 'boolean' and 'number'.2365Operator '<' cannot be applied to types 'boolean' and 'number'.grade < 70) {console .log ("D: Pass");} else {console .log ("F: Fail");}
bash
$ node grade.jsGrade: 95A: Pass$ node grade.jsGrade: 79A: Pass$ node grade.jsGrade: 42A: Pass$ node grade.jsGrade: chickenA: Pass
See Explanation
These are perfectly valid boolean conditions in javascript but do not evaluate intuitively. No matter what the value of grade is it will always evaluate to true even for non-numbers. Typescript would not let you do this.
Example 3
ts
const diceRoll = rollDice(100);if (diceRoll > 99) {mutallyAssuredDestruction();} else if (diceRoll > 98) {gainMoney();} else if (diceRoll > 80) {fortuneTellerPredictsDoom();} else if (diceRoll > 60) {npcTurnsTraitor();} else if (diceRoll > 40) {blackmailed();} else {runAway();}
ts
constdiceRoll =rollDice (100);if (diceRoll > 99) {mutallyAssuredDestruction ();} else if (diceRoll > 98) {Expected 1 arguments, but got 0.2554Expected 1 arguments, but got 0.(); gainMoney } else if (diceRoll > 80) {fortuneTellerPredictsDoom ();} else if (diceRoll > 60) {npcTurnsTraitor ();} else if (diceRoll > 40) {blackmailed ();} else {runAway ();}
text
$ node dice.jsYou run away!$ node dice.jsThe fortune teller predicts doom!$ node dice.jsYou've been blackmailed!$ node dice.jsYou run away!$ node dice.jsYou run away!
See Explanation
This is very much a real world problem. Here you cannot tell there is an issue with the code. In fact when running the code it seems to be working without any issues. But there is a bug that only happens one percent of the time because a function coming from somewhere else such as an external package or module is not being called correctly.
Typescript can tell when you misuse a function if it expects parameters that you are not passing or if you are misusing the returned value based on the return type.
Example 4
ts
const users = [{name: "John",role: {id: 1,name: "Admin",},}, {name: "Jane",}];for (let u of users) {console.log(`${u.name} is ${u.role.name}`);}
ts
constusers = [{name : "John",role : {id : 1,name : "Admin",},}, {name : "Jane",}];for (letu ofusers ) {'u.role' is possibly 'undefined'.18048'u.role' is possibly 'undefined'.console .log (`${u .name } is ${u .role .name }`);}
$ node null.jsJohn is AdminTypeError: Cannot read properties of undefined (reading 'name')at file:////src/null.js:15:38at ModuleJob.run (node:internal/modules/esm/module_job:218:25)at async ModuleLoader.import (node:internal/modules/esm/loader:329:24)at async loadESM (node:internal/process/esm_loader:28:7)at async handleMainPromise (node:internal/modules/run_main:113:12)
See Explanation
Typescript is pretty strict about null handling. If it thinks a value could possible be null or undefined then you are forced to null check the value first. This is a good practice that developers often overlook when writing plain javascript.
How do I use typescript?
Like previously said, javascript is valid typescript so if you are using javascript you dont need to change your syntax, just add //@ts-check
to the top of your JS files and if your editor support type checking it will start showing you types errors in your javascript.
This will not enable all the features of typescript including the additional syntax which we did not dig into in this post. So if you want to use typescript fully you will need to set up a compiler like tsc
or babel
to convert your typescript files to javascript. In the next post we will dive deeper into typescripts special syntax to take full advantage of the benefits typescript has to offer.